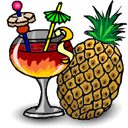
I have Google Analytics setup on my blog and I can see the keywords that have actually brought people to my site.... I find this interesting and this inspires me...If it's obscure, why not try to "guess" what the person was searching for and then do a post on that subject, if it's pretty straight forward,why not solve it..Today's keyword subject is : perl script handbrake windows
This one doesn't look too bad.
Per Handbrake.fr's site:
HandBrake is an open-sourced, GPL-Licensed,multiplatform, multithreaded, video transcoder, available for Mac OS X, Linux, and WindowsLooking at the documentation on handbrake's site, there is a command line interface, so we can use that to make any calls to the handbrake program.
The basic command we are going to use would be:
HandBrakeCLI -i source -o destinationSo we can immediately start with a perl script like this:
$source = "sourcefile";This will simply take whatever specified source file we have and output to the destination location.
$destination = $source . "_converted";
print `HandBrakeCLI -i $source -o $destination`;
But if that was all we wanted to do we wouldn't need a perl script would we?
How about if we have a list of files in a document (along with paths)?
We can open that document grab all the paths then simply do a mass conversion!
The final "guess" would simply grab all files of extension x and convert them and dump them in a folder. Again this isn't hard as well.
Lets start with this one, it shouldn't be too bad, of course we'll want to do some error checking, such as if we already have a converted filename of the same type then we definitely want to hold off on converting.
Regardless let's see what we can come up with real quick!
@file_list = <*.mpg *.avi *.vob>;Here we are grabbing a list of all the files we might want to convert, note that you can update that list to contain whatever extensions you might have/like to use. We also grab the list of converted files in there just to do some error checking (in case you leave all your files in the same folder), also the output type should match the type of files you are trying to convert to. We also should define our extension for later use!
@converted_list = <*.mp4>;
$extension = ".mp4";
Now it's a matter of rolling through each item in the filelist, so we'll start a for loop:
foreach $file (@file_list)Something to note is that we want to do a search on the filename in the converted files so in order to do that we'll need to break up the filename from the extension.
{
@filename = split(/./, $file);
$exists = 0;
}
Now inside this loop we'll want to do a quick check to see if the file we have a handle on is already listed
foreach $converted (@converted_files)So now we've done a check and if we have a file that our converted files contains.
{
if ($converted =~ m/@filename[0]/i)
{
$exists = 1;
}
$baseName = @filename[0];
}
What we can now do is make an if/then that will basically skip the file altogether if we see that $exists contains a 1!
if ($exists == 1)Something to remember:
{
print "File already converted!!";
}
else
{
$source = "$file";
$destination = $baseName . "_converted" . $extension;
print `HandBrakeCLI -i $source -o $destination`;
}
If you want to save to a separate folder I would recommend doing a check for whether that folder exists rather then grabbing a list of files in the current directory.Entire Script (small text...just copy paste):
@file_list = <*.mpg *.avi *.vob *.AVI>;The only difference between the above script and one that will open a document and pull all the filenames from it is that you would use the command:
@converted_list = <*.mp4>;
$extension = ".mp4";
foreach $file (@file_list)
{
@filename = split(/\./, $file);
$exists = 0;
foreach $converted (@converted_list)
{
if ($converted =~ m/@filename[0]/i)
{
$exists = 1;
}
$baseName = @filename[0];
print @filename[0] . "\n";
}
if ($exists == 1)
{
print "File already converted!!";
}
else
{
print "Converting";
$source = "$file";
$destination = $baseName . "_converted" . $extension;
print "Ready?\n";
$asdf = <>;
print `HandBrakeCLI -i $source -o $destination`;
}
}
open DATAFILE, "$inputfilename" or die "Missing $inputfilename file.\n"; open (DATAFILE, $inputfilename);The above would replace the line:
@file_list =;
close (DATAFILE);
@file_list = <*.mpg *.avi *.vob>;That's really the only difference!
Well let me know what you think in the comments and if you happen to have found this site looking for just this random post please let me know!!!